Marquee Tag animations are a powerful method for adding dynamic, moving content to a webpage. Traditionally implemented with the now-deprecated <marquee>
HTML tag, these animations allowed text or images to scroll across the screen. However, with advancements in web design, you can now use HTML, CSS, and JavaScript to create more responsive and customizable marquee effects. Marquee animations offer incredible versatility, serving various functions such as displaying sponsor logos, scrolling text announcements, and creating dynamic headers that captivate users. By incorporating these effects, websites gain a unique visual appeal and more engaging content.
We’ll go over how to create unique marquee effects step-by-step in this tutorial, utilizing JavaScript for extra interactivity, CSS for styling and animations, and HTML for structure. This tutorial will assist you in creating contemporary marquee animations that you can quickly implement and modify for your projects, regardless of your level of experience.
Table of Contents
What is a Marquee Animation?
Marquee animations are graphic effects that add a dynamic feel to a webpage by causing text and images to scroll or slide. When web design was just getting started, the. <marquee> Although the HTML tag had a lot of limitations, it was still used to implement these animations. It was less compatible with current web standards because it was non-standard, rigid, and offered few styling options.
Today’s method uses HTML, CSS, and JavaScript to create unique marquee effects. More control over the animations’ appearance, tempo, direction, and responsiveness is now possible. For example, the marquee’s structure can be specified in HTML and CSS, keyframes can be used to control movement, and JavaScript can interact with the animation or dynamically change the number of elements in response to user input.
With the switch to custom methods, marquee animations can now be customized to fit a wide range of web design requirements. Examples of these include news tickers, sponsor logos that scroll smoothly, and animated banners that draw in users without slowing down websites.
Why Make Custom Animations for Marquees?
Personalized marquee animations improve contemporary web design in a number of ways. Unlike the antiquated ones, they give developers total control over the styling, speed, and behavior of visually appealing scrolling elements. <marquee> affix. Personalized marquees are very versatile and can be utilized for a number of events.
As an illustration:
Sponsor Logo Display: A horizontal scroll of sponsor logos gives partnership or event pages a polished appearance. The speed, spacing, and size can all be changed to fit your design.
Scrolling Announcements: Suitable for urgent or revolving messages such as updates about events, special offers, or breaking news. Text can scroll across the page smoothly and without obstructing other design elements thanks to custom marquees.
Dynamic Headers: Custom marquee effects work incredibly well to create eye-catching, attention-grabbing headers that draw attention to and emphasize key information, such as promotions or featured content.
These animations have the added benefit of being responsive across various devices, which helps maintain websites interesting, contemporary, and interactive. Utilizing unique HTML, CSS, and JavaScript guarantees that your marquee blends in perfectly with the design of your website, giving visitors a polished and businesslike experience.
Setting Up the Environment HTML :
To get started with custom marquee animations, you’ll need to set up a basic HTML and CSS environment. Here’s a simple step-by-step guide:
- Step 1: Open Your Code Editor
Choose your preferred code editor like Visual Studio Code, Sublime Text, or Atom. This is where you will write and preview your code. - Step 2: Create an HTML File
Begin by creating a new HTML file (index.html). Inside this file, set up a basic HTML structure. Here’s what it looks like:
HTML
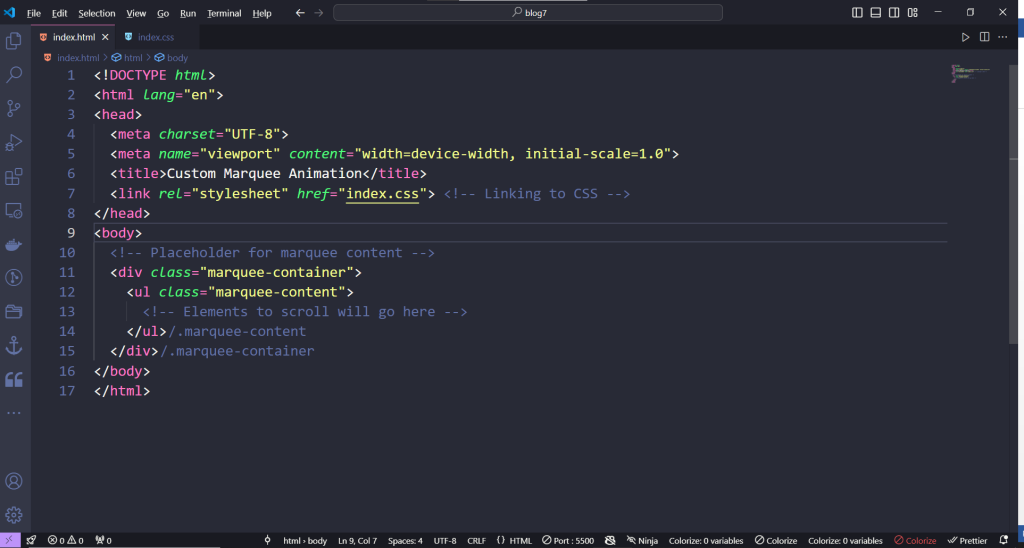
CSS
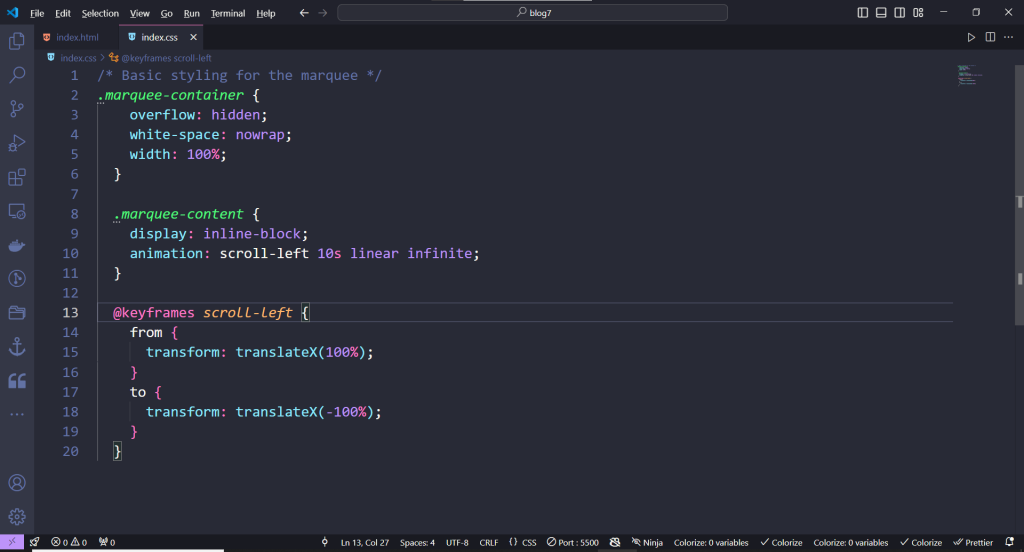
Explanation:
.marquee-container: ensures that the marquee stays within the visible area of the screen and hides overflowing content.
.marquee-content :applies the scrolling effect, which will move the items horizontally using the scroll-left animation.
@keyframes scroll-left : defines the animation that makes the content start off-screen on the right (translateX(100%)) and move left across the viewport until it’s off-screen on the left (translateX(-100%)).
By setting up this basic environment, you’re ready to start adding your marquee content and experimenting with different scrolling effects.
CODE PART
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Marquee Animation</title>
<link rel="stylesheet" href="index.css"> <!-- Linking to CSS -->
</head>
<body>
<!-- Placeholder for marquee content -->
<div class="marquee-container">
<ul class="marquee-content">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
</div>
</body>
</html>
CSS:
.marquee-container {
width: 100%;
overflow: hidden;
white-space: nowrap;
}
.marquee-content {
display: inline-block;
padding-left: 100%;
animation: scroll-left 10s linear infinite;
}
@keyframes scroll-left {
0% {
transform: translateX(100%);
}
100% {
transform: translateX(-100%);
}
}
Building a Basic Marquee Animation without javascript :
Let’s dive into creating a simple marquee animation using just HTML and CSS. Here’s how you can achieve a smooth scrolling effect without needing any JavaScript:
Step 1: HTML Structure:
We’ll use a simple structure with a div
container holding a list of items to be animated.
HTML
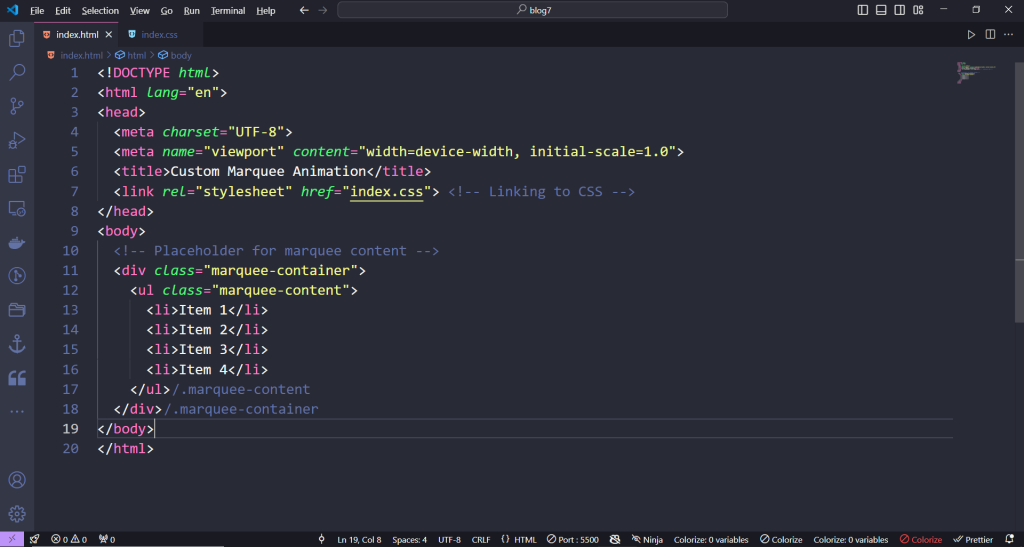
CSS
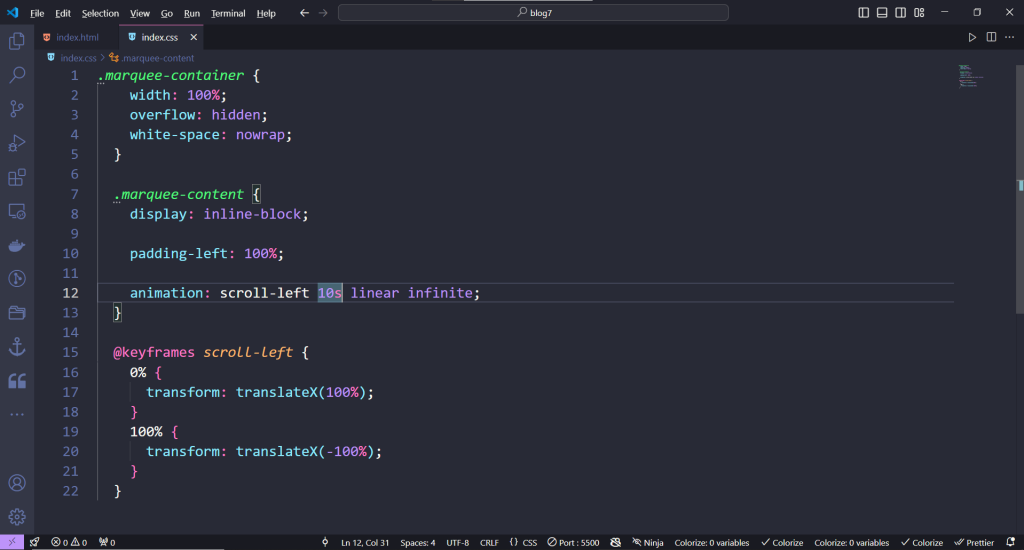
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Marquee Animation</title>
<link rel="stylesheet" href="index.css"> <!-- Linking to CSS -->
</head>
<body>
<!-- Placeholder for marquee content -->
<div class="marquee-container">
<ul class="marquee-content">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
</div>
</body>
</html>
CSS
.marquee-container {
width: 100%;
overflow: hidden;
white-space: nowrap;
}
.marquee-content {
display: inline-block;
padding-left: 100%;
animation: scroll-left 10s linear infinite;
}
@keyframes scroll-left {
0% {
transform: translateX(100%);
}
100% {
transform: translateX(-100%);
}
}
Explanation of CSS:
- .marquee-container {}: This sets the width of the container to 100% and hides any content that overflows using overflow: hidden. The white-space: nowrap; ensures that the content inside doesn’t wrap and stays in a single line for smooth scrolling.
- .marquee-content {}: The display: inline-block; ensures the list items line up horizontally, while padding-left: 100%; ensures the scrolling starts off-screen. The key part here is the animation: scroll-left 10s linear infinite; which applies an animation that scrolls the content from right to left over 10 seconds in an infinite loop.
- @keyframes scroll-left {}: This defines the animation. The content starts at translateX(100%); (off the right side of the screen) and moves to translateX(-100%); (off the left side of the screen).
Explanation of Code (Line by Line):
- .marquee-container { width: 100%; }: Ensures the container takes up the full width of the screen.
- overflow: hidden;: Hides any part of the content that moves outside the container.
- .marquee-content { display: inline-block; padding-left: 100%; }: Arranges the content in a single horizontal line and starts the animation from outside the visible area.
- animation: scroll-left 10s linear infinite;: The marquee will scroll continuously with a 10-second cycle.
- @keyframes scroll-left: Defines the exact movement, shifting content from right to left across the screen.
By using this approach, you create a smooth, simple marquee effect with pure HTML and CSS—no JavaScript required.
Building a Basic Marquee Animation with javascript :
Useing this javascript you can control the direction of the marquee tag and increase or decrease the speed of the movement of the object .
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Marquee Animation</title>
<link rel="stylesheet" href="index.css"> <!-- Linking to CSS -->
</head>
<body>
<!-- Placeholder for marquee content -->
<div class="marquee-container">
<ul class="marquee-content">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
</div>
<button id="speedUp">Speed Up</button>
<button id="slowDown">Slow Down</button>
<button id="changeDirection">Change Direction</button>
<script>
let marquee = document.querySelector('.marquee-content');
let speed = 10; // initial speed in seconds
function updateAnimation() {
marquee.style.animationDuration = `${speed}s`;
}
document.getElementById('speedUp').addEventListener('click', () => {
speed = Math.max(1, speed - 1);
updateAnimation();
});
document.getElementById('slowDown').addEventListener('click', () => {
speed += 1;
updateAnimation();
});
document.getElementById('changeDirection').addEventListener('click', () => {
marquee.style.animationDirection =
marquee.style.animationDirection === 'reverse' ? 'normal' : 'reverse';
});
updateAnimation();
</script>
</body>
</html>
Explanation of JavaScript:
let marquee = document.querySelector(‘.marquee-content’);: Selects the marquee element you want to control.
let speed = 10;: Sets the initial speed of the marquee (in seconds).
function updateAnimation(): A helper function that updates the animation’s speed by adjusting the animationDuration property based on the current speed value.
speedUp and slowDown buttons: These buttons increase or decrease the speed by modifying the animationDuration. The higher the number, the slower the marquee; the lower the number, the faster it moves.
changeDirection button: Toggles the animation direction between normal and reverse, changing the scrolling direction on the fly.
Step 2: Explanation (Line by Line):
let marquee = document.querySelector(‘.marquee-content’);: Grabs the marquee container for manipulation.
let speed = 10;: Sets an initial speed value of 10 seconds for the marquee’s scroll cycle.
function updateAnimation() { marquee.style.animationDuration = ${speed}s; }: Dynamically updates the speed of the marquee based on the current speed variable.
document.getElementById(‘speedUp’).addEventListener(‘click’, …);: On clicking the “Speed Up” button, the speed decreases (faster scroll), and the updateAnimation() function applies the new speed.
document.getElementById(‘changeDirection’).addEventListener(‘click’, …);: Toggles between normal and reverse directions using JavaScript.
This customization allows your marquee to be interactive, giving users control over speed and direction. By introducing JavaScript, you transform a basic marquee into a dynamic element that can be adjusted on the fly, offering better engagement and flexibility in web design.
Example 1: Basic image/text Marquee :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Custom Marquee Animation</title>
<link rel="stylesheet" href="index.css" />
<!-- Linking to CSS -->
</head>
<body>
<!-- Placeholder for marquee content -->
<div class="marquee-container">
<div class="image-marquee">
<img src="image1.jpg" alt="Logo 1" />
<img src="image2.jpg" alt="Logo 2" />
<img src="image3.jpg" alt="Logo 3" />
<!-- or you can place your text in form of heading or para etc by removeing the img :
like this :
<div class="basic-marquee">
<p>This is a simple scrolling marquee text!</p>
</div>
-->
</div>
</div>
</body>
</html>
CSS:
.image-marquee {
display: flex;
overflow: hidden;
width: 100%;
}
.image-marquee img {
width: 100px;
margin-right: 20px;
animation: scroll-images 15s linear infinite;
}
@keyframes scroll-images {
from { transform: translateX(100%); }
to { transform: translateX(-100%); }
}
Explanation (Line by Line):
- <div class=”image-marquee”>: A container for the scrolling images.
- .image-marquee { display: flex; overflow: hidden; }: Sets up a horizontal container for the images, with overflow hidden to create the scrolling effect.
- .image-marquee img { animation: scroll-images 15s linear infinite; }: Animates the images to scroll from right to left at a controlled speed.
- @keyframes scroll-images { from { transform: translateX(100%); } to { translateX(-100%); }}: Moves the images across the screen continuously.
Adjusting Speed and Direction:
- Speed: Change the 15s in the animation property to a shorter duration (e.g., 10s) for a faster scroll or longer duration (e.g., 20s) for a slower scroll.
- Direction: Reverse the translateX() values in the keyframes to scroll from left to right instead of right to left.
These examples demonstrate both a basic text marquee and an image marquee, offering versatility for your web design projects while keeping the code easy to understand and modify.
The Main Example ~blue_coders
1.) Logo Display :
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blue_coderz</title>
<link rel="stylesheet" href="index.css">
</head>
<body>
<div class="icon-marquee">
<ul class="icon-marquee-content">
<li><img src="./1.png" alt="Icon 1"></li>
<li><img src="./2.png" alt="Icon 2"></li>
<li><img src="./3.png" alt="Icon 3"></li>
<li><img src="./4.png" alt="Icon 4"></li>
<li><img src="./5.png" alt="Icon 5"></li>
<li><img src="./6.png" alt="Icon 6"></li>
<li><img src="./7.png" alt="Icon 7"></li>
<li><img src="./8.png" alt="Icon 8"></li>
</ul>
</div>
<script src="index.js"></script>
</body>
</html>
CSS:
/* General Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Montserrat', sans-serif;
background-color: white;
color: #111;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
/* Custom Marquee */
:root {
--icon-marquee-width: 80vw;
--icon-marquee-height: 20vh;
--icon-marquee-elements-displayed: 5;
--icon-marquee-element-width: calc(var(--icon-marquee-width) / var(--icon-marquee-elements-displayed));
--icon-marquee-animation-duration: calc(var(--icon-marquee-elements) * 3s);
}
.icon-marquee {
width: var(--icon-marquee-width);
height: var(--icon-marquee-height);
background-color: white;
overflow: hidden;
position: relative;
}
.icon-marquee:before, .icon-marquee:after {
position: absolute;
top: 0;
width: 12rem;
height: 100%;
content: "";
z-index: 1;
}
.icon-marquee:before {
left: 0;
background: linear-gradient(to right, #111 0%, transparent 100%);
}
.icon-marquee:after {
right: 0;
background: linear-gradient(to left, #111 0%, transparent 100%);
}
.icon-marquee-content {
list-style: none;
height: 100%;
display: flex;
animation: scroll var(--icon-marquee-animation-duration) linear infinite;
}
@keyframes scroll {
0% {
transform: translateX(0);
}
100% {
transform: translateX(calc(-1 * var(--icon-marquee-element-width) * var(--icon-marquee-elements)));
}
}
.icon-marquee-content li {
display: flex;
justify-content: center;
align-items: center;
flex-shrink: 0;
width: var(--icon-marquee-element-width);
max-height: 100%;
}
.icon-marquee-content li img {
width: 100%;
height: auto;
border: 2px solid #eee;
}
/* Responsive */
@media (max-width: 600px) {
:root {
--icon-marquee-width: 100vw;
--icon-marquee-height: 16vh;
--icon-marquee-elements-displayed: 3;
}
}
JavaScript :
const root = document.documentElement;
const iconMarqueeElementsDisplayed = getComputedStyle(root).getPropertyValue("--icon-marquee-elements-displayed");
const iconMarqueeContent = document.querySelector(".icon-marquee-content");
// Update the number of elements based on the number of icons
root.style.setProperty("--icon-marquee-elements", iconMarqueeContent.children.length);
// Clone the first few elements to make the marquee loop smoothly
for (let i = 0; i < iconMarqueeElementsDisplayed; i++) {
iconMarqueeContent.appendChild(iconMarqueeContent.children[i].cloneNode(true));
}
Explaination of the CSS:
/* General Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: ‘Montserrat’, sans-serif;
background-color: white;
color: #111;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
Reset Styles: Removes default padding and margins and sets box-sizing: border-box to include padding and border within the element’s total width/height.
Body Styles: Applies styles to the body, including setting the font, background color, and centering the content vertically and horizontally using flexbox.
/* Custom Marquee */
:root {
–icon-marquee-width: 80vw;
–icon-marquee-height: 20vh;
–icon-marquee-elements-displayed: 5;
–icon-marquee-element-width: calc(var(–icon-marquee-width) / var(–icon-marquee-elements-displayed));
–icon-marquee-animation-duration: calc(var(–icon-marquee-elements) * 3s);
}
Custom Properties (:root
): Defines CSS variables for marquee width, height, number of elements displayed, and animation duration. Using variables makes the code more flexible and easier to update.
.icon-marquee {
width: var(–icon-marquee-width);
height: var(–icon-marquee-height);
background-color: white;
overflow: hidden;
position: relative;
}
.icon-marquee:before, .icon-marquee:after {
position: absolute;
top: 0;
width: 12rem;
height: 100%;
content: “”;
z-index: 1;
}
.icon-marquee: Sets the size and background for the marquee container and hides overflowing content using overflow: hidden.
:before, :after: These pseudo-elements create a gradient effect on either side of the marquee to smoothly blend the icons as they scroll in and out of view.
.icon-marquee-content {
list-style: none;
height: 100%;
display: flex;
animation: scroll var(–icon-marquee-animation-duration) linear infinite;
}
@keyframes scroll {
0% {
transform: translateX(0);
}
100% {
transform: translateX(calc(-1 * var(–icon-marquee-element-width) * var(–icon-marquee-elements)));
}
}
@keyframes scroll
: Defines the animation for the marquee, moving the content from right to left by translating it along the X-axis.
List Item Styles: Ensures that each image is properly centered and that the items do not shrink, keeping the size consistent.
JavaScript Explanation :
const root = document.documentElement;
const iconMarqueeElementsDisplayed = getComputedStyle(root).getPropertyValue(“–icon-marquee-elements-displayed”);
const iconMarqueeContent = document.querySelector(“.icon-marquee-content”);
// Update the number of elements based on the number of icons
root.style.setProperty(“–icon-marquee-elements”, iconMarqueeContent.children.length);
· Root Element: Accesses the root element (:root) and retrieves the number of elements to display from the CSS custom property.
· Updating Elements: Updates the –icon-marquee-elements property based on the number of child elements inside .icon-marquee-content.
// Clone the first few elements to make the marquee loop smoothly
for (let i = 0; i < iconMarqueeElementsDisplayed; i++) {
iconMarqueeContent.appendChild(iconMarqueeContent.children[i].cloneNode(true));
}
- Cloning Elements: Clones the first few images and appends them to the end of the marquee to ensure a smooth and continuous loop.
By breaking down the code line by line, this explanation ensures that your users understand how each part works and how they can customize it for their own needs.
Explanation of the Michael Jackson GIF Marquee Example :
Code Part
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blue_coders_</title>
<link rel="stylesheet" href="index.css">
</head>
<body>
<marquee class="jackson" direction="left">
<img src="https://media.giphy.com/media/EDZP0UCtxiRQQ/giphy.gif" alt="">
<img src="" alt="">
<img src="" alt="">
<img src="" alt="">
<img src="" alt="">
<img src="./car-1803_256.gif" id="car" alt="">
</marquee>
</body>
</html>
CSS
:root {
--duration: 4s;
}
body {
padding: 0;
margin: 0;
background-color: white; /* Set background to white */
display: flex;
align-items: center; /* Vertically center */
justify-content: center; /* Horizontally center */
min-height: 100vh; /* Ensure the body takes the full height of the viewport */
}
.jackson {
animation: color var(--duration) linear infinite;
width: 100%;
background-color: white; /* Ensure the marquee background is white */
overflow: hidden; /* Prevent any overflow */
display: flex;
align-items: center; /* Center the content vertically */
}
#car {
position: relative;
top: 82px;
margin-left: auto;
}
· <marquee class="jackson" direction="left">
: This creates the marquee effect, making the content scroll continuously from right to left.
- The
class="jackson"
will be styled in the CSS. - The
direction="left"
attribute ensures that the images scroll from right to left.
· <img src="..." alt="">
: Displays multiple images inside the marquee. One is a Michael Jackson dancing GIF, while the others can be set up as needed.
· The last image <img src="./car-1803_256.gif" id="car" alt="">
: Adds a car GIF to the marquee and gives it a unique ID for styling.
Css explanation:
· :root { –duration: 4s; }: This defines a CSS variable –duration with a value of 4s, which controls the speed of the animation.
· body: Ensures the content is centered both vertically and horizontally within the viewport.
- background-color: white;: The body has a white background to make the marquee stand out.
- display: flex; align-items: center; justify-content: center;: Flexbox is used to center the marquee.
· .jackson {}: Applies styles to the marquee.
- animation: color var(–duration) linear infinite;: Animates the marquee smoothly.
- width: 100%;: Ensures the marquee spans the entire width of the page.
- overflow: hidden;: Ensures no content spills out of the marquee area.
- display: flex; align-items: center;: Centers the images vertically within the marquee.
· #car {}: Styles the car GIF specifically.
- position: relative; top: 82px;: Positions the car image so that it appears slightly below the other images.
- margin-left: auto;: This pushes the car to the right side of the marquee.
Explanation of the Netflix kind of Marquee Example :
Code Part :
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>blue_coderz</title>
<link rel="shortcut icon" href="./profile3.png" type="image/x-icon">
<link rel="stylesheet" href="index.css" />
</head>
<body>
<div class="container">
<div class="text">
<div class="txt">
<h1 >Because </h1>
<h1 >you love </h1>
<h1 >movies.</h1>
</div>
</div>
<div class="box">
<div class="slider">
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
</div>
<div class="slider">
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
</div>
<div class="slider">
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
</div>
<div class="slider1">
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
<div class="slide-track">
<img src="./3.jpg" alt="Movie 3" />
<img src="./7.jpg" alt="Movie 7" />
<img src="./1.jpg" alt="Movie 1" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./4.jpg" alt="Movie 4" />
<img src="./2.jpg" alt="Movie 2" />
<img src="./11.jpg" alt="Movie 11" />
<img src="./5.jpg" alt="Movie 5" />
<img src="./9.jpg" alt="Movie 9" />
<img src="./8.jpg" alt="Movie 8" />
<img src="./3.jpg" alt="Movie 3" />
<!-- Duplicates for smooth loop -->
<img src="./7.jpg" alt="Movie 7" />
<img src="./10.jpg" alt="Movie 10" />
<img src="./6.jpg" alt="Movie 6" />
<img src="./1.jpg" alt="Movie 1" />
</div>
</div>
</div>
</div>
</body>
</html>
CSS
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
background-color: black;
font-family: Arial, sans-serif;
color: white;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
overflow: hidden;
}
.container {
display: flex;
flex-direction: row;
align-items: center;
position: relative;
width: 100%;
;
}
.txt {
position: relative;
top: 117px;
padding-left: 41px;
}
.txt h1{
font-size: 144px;
}
.text {
background-image: linear-gradient(to right, rgb(11, 11, 11) , rgba(28, 28, 27, 0.875));
position:absolute;
z-index: 2;
width: 800px;
height: 700px;
}
h1 {
font-size: 4em;
color: snow;
}
.slider {
width: 70vw;
height: 350px;
overflow: hidden;
position: absolute;
right: 0;
}
.slide-track {
display: flex;
width: calc(250px * 14); /* Adjust based on number of images */
animation: scroll 20s linear infinite;
transform: translateZ(-150px) rotateY(-10deg); /* Slight curved effect */
}
.slide-track img {
width: 250px;
height: 350px;
object-fit: cover;
margin: 10px;
}
.box {
display: flex;
flex-direction: row;
transform:rotate3d(0,1,0,45deg);
justify-content: space-evenly;
filter: grayscale(50%);
}
@keyframes scroll {
0% {
transform: translateX(0) translateZ(-150px) rotateY(-10deg); /* Start at normal position */
}
100% {
transform: translateX(calc(-250px * 7)) translateZ(-150px) rotateY(-10deg); /* Move across the screen */
}
}
detailed step-by-step explanation of the HTML code:
1. <!DOCTYPE html>
- This declaration defines the document as an HTML5 document.
2. <html lang="en">
- The
<html>
tag marks the beginning of the HTML document. lang="en"
specifies that the language of the document is English.
3. <head>
- The
<head>
tag contains metadata and links to stylesheets or scripts for the webpage.
4. <meta charset="UTF-8" />
- Sets the character encoding to UTF-8, ensuring the webpage can display a wide range of characters.
5. <meta name="viewport" content="width=device-width, initial-scale=1.0" />
- Ensures that the webpage is responsive.
- It sets the width of the page to match the screen width and initial zoom level.
6. <title>blue_coderz</title>
- Defines the title of the webpage, which appears on the browser tab.
7. <link rel="shortcut icon" href="./profile3.png" type="image/x-icon">
- Links a favicon (shortcut icon) to the webpage, using the image
profile3.png
.
8. <link rel="stylesheet" href="index.css" />
- Links an external CSS stylesheet called
index.css
to style the webpage.
9. <body>
- The
<body>
tag marks the start of the content that will be visible on the webpage.
10. <div class="container">
- Creates a container
<div>
, which is likely used to group and style inner content.
11. <div class="text">
- A
<div>
element with the classtext
, probably used to style text elements inside it.
12. <div class="txt">
- Another
<div>
element, with the classtxt
, used to group the<h1>
elements.
13. <h1>Because</h1>
- A heading element displaying the text “Because.”
14. <h1>you love</h1>
- A heading element displaying the text “you love.”
15. <h1>movies.</h1>
- A heading element displaying the text “movies.” Together, these headings create the sentence “Because you love movies.”
16. <div class="box">
- A
<div>
element with the classbox
, likely used to style and structure the content within it.
17. <div class="slider">
- This
slider
<div>
is used to contain the sliding image content.
18. <div class="slide-track">
- The
slide-track
<div>
holds a series of images that will move or slide, giving the slider effect.
19. <img src="./3.jpg" alt="Movie 3" />
- An image element that loads
3.jpg
as the source file. Thealt
text describes the image as “Movie 3.”
20. Repeated <img>
tags
- This series of
<img>
tags loads different movie images (e.g.,7.jpg
,1.jpg
, etc.). The images are grouped into three separate slide tracks for smoother looping. - Duplicate images are included after the original series to ensure a continuous loop in the slider.
21. Repetition of Slide Tracks
- The next few
div class="slide-track"
elements repeat the same structure to ensure a smooth, visually appealing image slider with multiple lanes.
Each slider and slide track is likely styled with CSS and animated with JavaScript (if needed) to create a looping, continuous carousel effect. You’ll notice multiple slide-track
divs; these may represent different tracks or lanes of images sliding in a continuous loop, mimicking a multi-layered slider.
This HTML structure forms the base of a visually dynamic webpage, with the content grouped and structured logically to display multiple images in a continuous loop alongside some heading text. The actual slider behavior will be controlled by CSS animations or JavaScript.
detailed explanation of the CSS for the image slider:
- Slider Container:
The.slider-container
class defines the overall space where the slider will be visible. Thewidth
andheight
properties set the size of the slider, ensuring it fits into your webpage layout. Theoverflow: hidden
property is crucial here; it hides any parts of the slider that extend beyond the container’s dimensions. This is what gives the impression of an endless sliding effect since images that slide out of view are clipped and no longer visible. - Slider:
The.slider
class is responsible for holding all the images. By settingdisplay: flex
, it arranges the images in a horizontal row rather than stacking them vertically (the default layout for block elements). Theanimation
property is where the sliding action happens. It assigns an animation calledscroll
that moves the images across the screen. Theinfinite
keyword ensures the animation never stops—it loops continuously. You can control the speed of the slide with theduration
(like10s
,15s
, etc.). - Image Styling:
The.slider img
class styles the individual images inside the slider. Thewidth
property ensures that the images fit within their allotted space. Setting it to100%
means the images will take up the entire width of their parent container, keeping them responsive. Alternatively, you can set the width to a fixed size like300px
if you want all the images to have the same dimensions. You can also add padding or margins if you want space between the images. - Keyframes for Animation:
The@keyframes scroll
defines the animation steps for the sliding effect. It works by adjusting thetransform: translateX()
property, which shifts the entire row of images horizontally. At0%
, the slider starts in its original position, and by100%
, the slider has moved to the left by a set percentage or pixel value. This creates the effect of the images continuously moving from right to left. The speed and smoothness of the slide can be fine-tuned by adjusting the keyframe values and theanimation
duration. Thelinear
timing function ensures that the slider moves at a constant speed, without any pauses or slow-downs.
This CSS setup ensures that the images in the slider flow smoothly across the container, creating a continuous scrolling effect that’s visually engaging.